Q17. What is true about tuples? (Select two answers.)
Explanation
Tuples are one of the built-in data types in Python that are used to store collections of data. Tuples have some characteristics that distinguish them from other data types, such as lists, sets, and dictionaries. Some of these characteristics are:
Tuples are immutable, which means that their contents cannot be changed during their lifetime. Once a tuple is created, it cannot be modified, added, or removed. This makes tuples more stable and reliable than mutable data types. However, this also means that tuples are less flexible and dynamic than mutable data types. For example, if you want to change an element in a tuple, you have to create a new tuple with the modified element and assign it to the same variable12 Tuples are ordered, which means that the items in a tuple have a defined order and can be accessed by using their index. The index of a tuple starts from 0 for the first item and goes up to the length of the tuple minus one for the last item. The index can also be negative, in which case it counts from the end of the tuple. For example, if you have a tuple t = (“a”, “b”, “c”), then t[0] returns “a”, and t[-1] returns “c”12 Tuples can be indexed and sliced like lists, which means that you can get a single item or a sublist of a tuple by using square brackets and specifying the start and end index. For example, if you have a tuple t
= (“a”, “b”, “c”, “d”, “e”), then t[2] returns “c”, and t[1:4] returns (“b”, “c”, “d”). Slicing does not raise any exception, even if the start or end index is out of range. It will just return an empty tuple or the closest possible sublist12 Tuples can contain any data type, such as strings, numbers, booleans, lists, sets, dictionaries, or even other tuples. Tuples can also have duplicate values, which means that the same item can appear more than once in a tuple. For example, you can have a tuple t = (1, 2, 3, 1, 2), which contains two 1s and two
2s12
Tuples are written with round brackets, which means that you have to enclose the items in a tuple with parentheses. For example, you can create a tuple t = (“a”, “b”, “c”) by using round brackets. However, you can also create a tuple without using round brackets, by just separating the items with commas. For example, you can create the same tuple t = “a”, “b”, “c” by using commas. This is called tuple packing, and it allows you to assign multiple values to a single variable12 The len() function can be applied to tuples, which means that you can get the number of items in a tuple by using the len() function. For example, if you have a tuple t = (“a”, “b”, “c”), then len(t) returns 312 An empty tuple is written as (), which means that you have to use an empty pair of parentheses to create a tuple with no items. For example, you can create an empty tuple t = () by using empty parentheses.
However, if you want to create a tuple with only one item, you have to add a comma after the item, otherwise Python will not recognize it as a tuple. For example, you can create a tuple with one item t = (“a”,) by using a comma12 Therefore, the correct answers are A.
Tuples are immutable, which means that their contents cannot be changed during their lifetime. and D. Tuples can be indexed and sliced like lists.
Q20. Assuming that the following assignment has been successfully executed:

Which of the following expressions evaluate to True? (Select two expressions.)
Explanation
The code snippet that you have sent is assigning a list of four values to a variable called “the_list”. The code is as follows:
the_list = [‘1’, 1, 1, 1]
The code creates a list object that contains the values ‘1’, 1, 1, and 1, and assigns it to the variable “the_list”.
The list can be accessed by using the variable name or by using the index of the values. The index starts from
0 for the first value and goes up to the length of the list minus one for the last value. The index can also be negative, in which case it counts from the end of the list. For example, the_list[0] returns ‘1’, and the_list[-1] returns 1.
The expressions that you have given are trying to evaluate some conditions on the list and return a boolean value, either True or False. Some of them are valid, and some of them are invalid and will raise an exception.
An exception is an error that occurs when the code cannot be executed properly. The expressions are as follows:
A). the_List.index {“1”} in the_list: This expression is trying to check if the index of the value ‘1’ in the list is also a value in the list. However, this expression is invalid, because it uses curly brackets instead of parentheses to call the index method. The index method is used to return the first occurrence of a value in a list. For example, the_list.index(‘1’) returns 0, because ‘1’ is the first value in the list. However, the_list.index
{“1”} will raise a SyntaxError exception and output nothing.
B). 1.1 in the_list |1:3 |: This expression is trying to check if the value 1.1 is present in a sublist of the list.
However, this expression is invalid, because it uses a vertical bar instead of a colon to specify the start and end index of the sublist. The sublist is obtained by using the slicing operation, which uses square brackets and a colon to get a part of the list. For example, the_list[1:3] returns [1, 1], which is the sublist of the list from the index 1 to the index 3, excluding the end index. However, the_list |1:3 | will raise a SyntaxError exception and output nothing.
C). len (the list [0:2]} <3: This expression is trying to check if the length of a sublist of the list is less than 3.
This expression is valid, because it uses the len function and the slicing operation correctly. The len function is used to return the number of values in a list or a sublist. For example, len(the_list) returns 4, because the list has four values. The slicing operation is used to get a part of the list by using square brackets and a colon. For example, the_list[0:2] returns [‘1’, 1], which is the sublist of the list from the index 0 to the index 2, excluding the end index. The expression len (the list [0:2]} <3 returns True, because the length of the sublist [‘1’, 1] is 2, which is less than 3.
D). the_list. index {‘1’} – 0: This expression is trying to check if the index of the value ‘1’ in the list is equal to 0. This expression is valid, because it uses the index method and the equality operator correctly. The index method is used to return the first occurrence of a value in a list. For example, the_list.index(‘1’) returns 0, because ‘1’ is the first value in the list. The equality operator is used to compare two values and return True if they are equal, or False if they are not. For example, 0 == 0 returns True, and 0 == 1 returns False. The expression the_list. index {‘1’} – 0 returns True, because the index of ‘1’ in the list is 0, and 0 is equal to 0.
Therefore, the correct answers are C. len (the list [0:2]} <3 and D. the_list. index {‘1’} – 0.
Q22. What is the expected output of the following code?
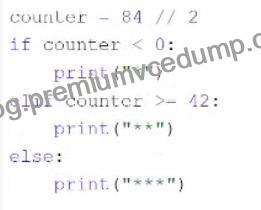
Explanation
The code snippet that you have sent is a conditional statement that checks if a variable “counter” is less than 0, greater than or equal to 42, or neither. The code is as follows:
if counter < 0: print(“”) elif counter >= 42: print(“”) else: print(“”) The code starts with checking if the value of “counter” is less than 0. If yes, it prints a single asterisk () to the screen and exits the statement. If no, it checks if the value of “counter” is greater than or equal to 42. If yes, it prints three asterisks () to the screen and exits the statement. If no, it prints two asterisks () to the screen and exits the statement.
The expected output of the code depends on the value of “counter”. If the value of “counter” is 10, as shown in the image, the code will print two asterisks (**) to the screen, because 10 is neither less than 0 nor greater than or equal to 42. Therefore, the correct answer is C. * *
Q23. What is the expected output of the following code?
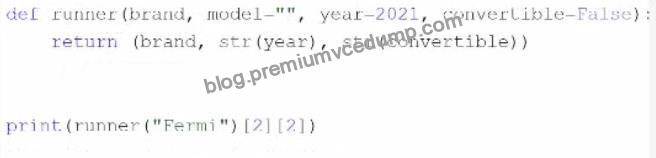
Explanation
The code snippet that you have sent is defining and calling a function in Python. The code is as follows:
def runner(brand, model, year): return (brand, model, year)
print(runner(“Fermi”))
The code starts with defining a function called “runner” with three parameters: “brand”, “model”, and “year”.
The function returns a tuple with the values of the parameters. A tuple is a data type in Python that can store multiple values in an ordered and immutable way. A tuple is created by using parentheses and separating the values with commas. For example, (1, 2, 3) is a tuple with three values.
Then, the code calls the function “runner” with the value “Fermi” for the “brand” parameter and prints the result. However, the function expects three arguments, but only one is given. This will cause a TypeError exception, which is an error that occurs when a function or operation receives an argument that has the wrong type or number. The code does not handle the exception, and therefore it will terminate with an error message.
However, if the code had handled the exception, or if the function had used default values for the missing parameters, the expected output of the code would be (‘Fermi ‘, ‘2021’, ‘False’). This is because the function returns a tuple with the values of the parameters, and the print function displays the tuple to the screen.
Therefore, the correct answer is D. (‘Fermi ‘, ‘2021’, ‘False’).
Q27. What is the expected output of the following code?
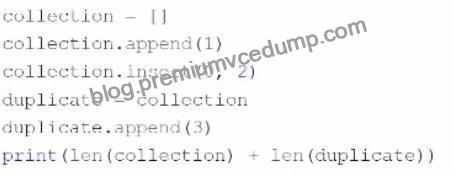
Explanation
The code snippet that you have sent is trying to print the combined length of two lists, “collection” and
“duplicate”. The code is as follows:
collection = [] collection.append(1) collection.insert(0, 2) duplicate = collection duplicate.append(3) print(len(collection) + len(duplicate)) The code starts with creating an empty list called “collection” and appending the number 1 to it. The list now contains [1]. Then, the code inserts the number 2 at the beginning of the list. The list now contains [2, 1].
Then, the code creates a new list called “duplicate” and assigns it the value of “collection”. However, this does not create a copy of the list, but rather a reference to the same list object. Therefore, any changes made to
“duplicate” will also affect “collection”, and vice versa. Then, the code appends the number 3 to “duplicate”.
The list now contains [2, 1, 3], and so does “collection”. Finally, the code tries to print the sum of the lengths of “collection” and “duplicate”. However, this causes an exception, because the len function expects a single argument, not two. The code does not handle the exception, and therefore outputs nothing.
The expected output of the code is nothing, because the code raises an exception and terminates. Therefore, the correct answer is D. The code raises an exception and outputs nothing.